
题目描述
Alice 把 n
个气球排列在一根绳子上。给你一个下标从 0 开始的字符串 colors
,其中 colors[i]
是第 i
个气球的颜色。
Alice 想要把绳子装扮成 五颜六色的 ,且她不希望两个连续的气球涂着相同的颜色,所以她喊来 Bob 帮忙。Bob 可以从绳子上移除一些气球使绳子变成 彩色 。给你一个 下标从 0 开始 的整数数组 neededTime
,其中 neededTime[i]
是 Bob 从绳子上移除第 i
个气球需要的时间(以秒为单位)。
返回 Bob 使绳子变成 彩色 需要的 最少时间 。
示例 1:
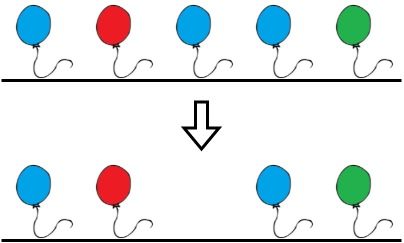
输入:colors = "abaac", neededTime = [1,2,3,4,5]
输出:3
解释:在上图中,'a' 是蓝色,'b' 是红色且 'c' 是绿色。
Bob 可以移除下标 2 的蓝色气球。这将花费 3 秒。
移除后,不存在两个连续的气球涂着相同的颜色。总时间 = 3 。
示例 2:
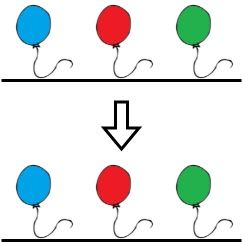
输入:colors = "abc", neededTime = [1,2,3]
输出:0
解释:绳子已经是彩色的,Bob 不需要从绳子上移除任何气球。
示例 3:
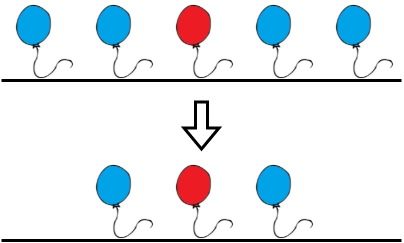
输入:colors = "aabaa", neededTime = [1,2,3,4,1]
输出:2
解释:Bob 会移除下标 0 和下标 4 处的气球。这两个气球各需要 1 秒来移除。
移除后,不存在两个连续的气球涂着相同的颜色。总时间 = 1 + 1 = 2 。
提示:
n == colors.length == neededTime.length
1 <= n <= 105
1 <= neededTime[i] <= 104
colors
仅由小写英文字母组成
解法
方法一:双指针 + 贪心
我们可以用双指针指向当前连续相同颜色的气球的首尾,然后计算出当前连续相同颜色的气球的总时间 $s$,以及最大的时间 $mx$。如果当前连续相同颜色的气球个数大于 $1$,那么我们可以贪心地选择保留时间最大的气球,然后移除其它相同颜色的气球,耗时 $s - mx$,累加到答案中。接下来继续遍历,直到遍历完所有气球。
时间复杂度 $O(n)$,空间复杂度 $O(1)$。其中 $n$ 为气球的个数。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16 | class Solution:
def minCost(self, colors: str, neededTime: List[int]) -> int:
ans = i = 0
n = len(colors)
while i < n:
j = i
s = mx = 0
while j < n and colors[j] == colors[i]:
s += neededTime[j]
if mx < neededTime[j]:
mx = neededTime[j]
j += 1
if j - i > 1:
ans += s - mx
i = j
return ans
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19 | class Solution {
public int minCost(String colors, int[] neededTime) {
int ans = 0;
int n = neededTime.length;
for (int i = 0, j = 0; i < n; i = j) {
j = i;
int s = 0, mx = 0;
while (j < n && colors.charAt(j) == colors.charAt(i)) {
s += neededTime[j];
mx = Math.max(mx, neededTime[j]);
++j;
}
if (j - i > 1) {
ans += s - mx;
}
}
return ans;
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20 | class Solution {
public:
int minCost(string colors, vector<int>& neededTime) {
int ans = 0;
int n = colors.size();
for (int i = 0, j = 0; i < n; i = j) {
j = i;
int s = 0, mx = 0;
while (j < n && colors[j] == colors[i]) {
s += neededTime[j];
mx = max(mx, neededTime[j]);
++j;
}
if (j - i > 1) {
ans += s - mx;
}
}
return ans;
}
};
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18 | func minCost(colors string, neededTime []int) (ans int) {
n := len(colors)
for i, j := 0, 0; i < n; i = j {
j = i
s, mx := 0, 0
for j < n && colors[j] == colors[i] {
s += neededTime[j]
if mx < neededTime[j] {
mx = neededTime[j]
}
j++
}
if j-i > 1 {
ans += s - mx
}
}
return
}
|