
题目描述
给定一个长度为 n
的整数数组 height
。有 n
条垂线,第 i
条线的两个端点是 (i, 0)
和 (i, height[i])
。
找出其中的两条线,使得它们与 x
轴共同构成的容器可以容纳最多的水。
返回容器可以储存的最大水量。
说明:你不能倾斜容器。
示例 1:
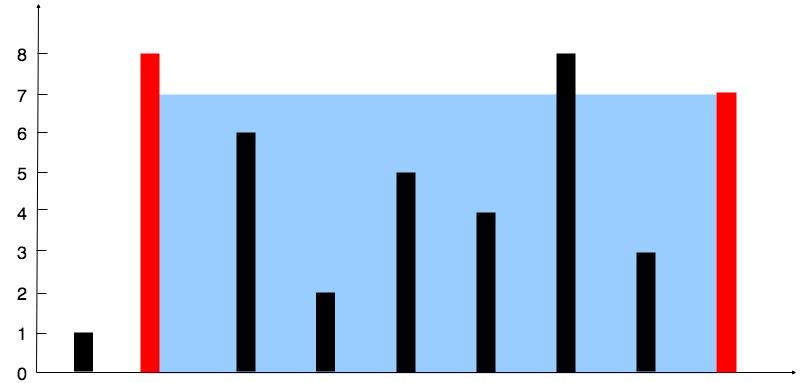
输入:[1,8,6,2,5,4,8,3,7]
输出:49
解释:图中垂直线代表输入数组 [1,8,6,2,5,4,8,3,7]。在此情况下,容器能够容纳水(表示为蓝色部分)的最大值为 49。
示例 2:
输入:height = [1,1]
输出:1
提示:
n == height.length
2 <= n <= 105
0 <= height[i] <= 104
解法
方法一:双指针
一开始,我们考虑相距最远的两个柱子所能容纳水的容量。水的宽度是两根柱子之间的距离,而水的高度取决于两根柱子之间较短的那个。
当前柱子是最两侧的柱子,水的宽度最大,其他的组合,水的宽度都比这个小。不妨假设左侧柱子的高度小于等于右侧柱子的高度,那么水的高度就是左侧柱子的高度。如果我们移动右侧柱子,那么水的宽度就减小了,而水的高度却不会增加,因此水的容量一定减少。所以我们移动左侧柱子,更新最大容量。
循环此过程,直到两个柱子相遇。
时间复杂度 $O(n)$,其中 $n$ 是数组 height
的长度。空间复杂度 $O(1)$。
1
2
3
4
5
6
7
8
9
10
11
12 | class Solution:
def maxArea(self, height: List[int]) -> int:
i, j = 0, len(height) - 1
ans = 0
while i < j:
t = (j - i) * min(height[i], height[j])
ans = max(ans, t)
if height[i] < height[j]:
i += 1
else:
j -= 1
return ans
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16 | class Solution {
public int maxArea(int[] height) {
int i = 0, j = height.length - 1;
int ans = 0;
while (i < j) {
int t = Math.min(height[i], height[j]) * (j - i);
ans = Math.max(ans, t);
if (height[i] < height[j]) {
++i;
} else {
--j;
}
}
return ans;
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17 | class Solution {
public:
int maxArea(vector<int>& height) {
int i = 0, j = height.size() - 1;
int ans = 0;
while (i < j) {
int t = min(height[i], height[j]) * (j - i);
ans = max(ans, t);
if (height[i] < height[j]) {
++i;
} else {
--j;
}
}
return ans;
}
};
|
1
2
3
4
5
6
7
8
9
10
11
12
13 | func maxArea(height []int) (ans int) {
i, j := 0, len(height)-1
for i < j {
t := min(height[i], height[j]) * (j - i)
ans = max(ans, t)
if height[i] < height[j] {
i++
} else {
j--
}
}
return
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15 | function maxArea(height: number[]): number {
let i = 0;
let j = height.length - 1;
let ans = 0;
while (i < j) {
const t = Math.min(height[i], height[j]) * (j - i);
ans = Math.max(ans, t);
if (height[i] < height[j]) {
++i;
} else {
--j;
}
}
return ans;
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16 | impl Solution {
pub fn max_area(height: Vec<i32>) -> i32 {
let mut i = 0;
let mut j = height.len() - 1;
let mut ans = 0;
while i < j {
ans = ans.max(height[i].min(height[j]) * ((j - i) as i32));
if height[i] <= height[j] {
i += 1;
} else {
j -= 1;
}
}
ans
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19 | /**
* @param {number[]} height
* @return {number}
*/
var maxArea = function (height) {
let i = 0;
let j = height.length - 1;
let ans = 0;
while (i < j) {
const t = Math.min(height[i], height[j]) * (j - i);
ans = Math.max(ans, t);
if (height[i] < height[j]) {
++i;
} else {
--j;
}
}
return ans;
};
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16 | public class Solution {
public int MaxArea(int[] height) {
int i = 0, j = height.Length - 1;
int ans = 0;
while (i < j) {
int t = Math.Min(height[i], height[j]) * (j - i);
ans = Math.Max(ans, t);
if (height[i] < height[j]) {
++i;
} else {
--j;
}
}
return ans;
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21 | class Solution {
/**
* @param Integer[] $height
* @return Integer
*/
function maxArea($height) {
$i = 0;
$j = count($height) - 1;
$ans = 0;
while ($i < $j) {
$t = min($height[$i], $height[$j]) * ($j - $i);
$ans = max($ans, $t);
if ($height[$i] < $height[$j]) {
++$i;
} else {
--$j;
}
}
return $ans;
}
}
|