题目描述
树可以看成是一个连通且 无环 的 无向 图。
给定往一棵 n
个节点 (节点值 1~n
) 的树中添加一条边后的图。添加的边的两个顶点包含在 1
到 n
中间,且这条附加的边不属于树中已存在的边。图的信息记录于长度为 n
的二维数组 edges
,edges[i] = [ai, bi]
表示图中在 ai
和 bi
之间存在一条边。
请找出一条可以删去的边,删除后可使得剩余部分是一个有着 n
个节点的树。如果有多个答案,则返回数组 edges
中最后出现的边。
示例 1:
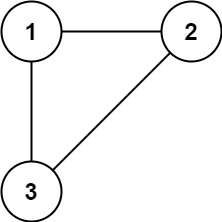
输入: edges = [[1,2],[1,3],[2,3]]
输出: [2,3]
示例 2:
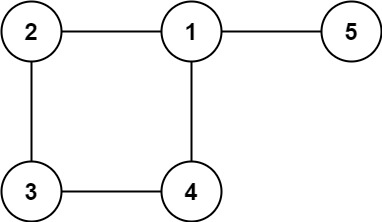
输入: edges = [[1,2],[2,3],[3,4],[1,4],[1,5]]
输出: [1,4]
提示:
n == edges.length
3 <= n <= 1000
edges[i].length == 2
1 <= ai < bi <= edges.length
ai != bi
edges
中无重复元素
- 给定的图是连通的
注意:本题与主站 684 题相同: https://leetcode.cn/problems/redundant-connection/
解法
方法一
1
2
3
4
5
6
7
8
9
10
11
12
13 | class Solution:
def findRedundantConnection(self, edges: List[List[int]]) -> List[int]:
def find(x):
if p[x] != x:
p[x] = find(p[x])
return p[x]
p = list(range(1010))
for a, b in edges:
if find(a) == find(b):
return [a, b]
p[find(a)] = find(b)
return []
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25 | class Solution {
private int[] p;
public int[] findRedundantConnection(int[][] edges) {
p = new int[1010];
for (int i = 0; i < p.length; ++i) {
p[i] = i;
}
for (int[] e : edges) {
int a = e[0], b = e[1];
if (find(a) == find(b)) {
return e;
}
p[find(a)] = find(b);
}
return null;
}
private int find(int x) {
if (p[x] != x) {
p[x] = find(p[x]);
}
return p[x];
}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20 | class Solution {
public:
vector<int> p;
vector<int> findRedundantConnection(vector<vector<int>>& edges) {
p.resize(1010);
for (int i = 0; i < p.size(); ++i) p[i] = i;
for (auto& e : edges) {
int a = e[0], b = e[1];
if (find(a) == find(b)) return e;
p[find(a)] = find(b);
}
return {};
}
int find(int x) {
if (p[x] != x) p[x] = find(p[x]);
return p[x];
}
};
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21 | func findRedundantConnection(edges [][]int) []int {
p := make([]int, 1010)
for i := range p {
p[i] = i
}
var find func(x int) int
find = func(x int) int {
if p[x] != x {
p[x] = find(p[x])
}
return p[x]
}
for _, e := range edges {
a, b := e[0], e[1]
if find(a) == find(b) {
return []int{a, b}
}
p[find(a)] = find(b)
}
return []int{}
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25 | class Solution {
private var parent: [Int] = []
func findRedundantConnection(_ edges: [[Int]]) -> [Int] {
parent = Array(0..<1010)
for edge in edges {
let a = edge[0]
let b = edge[1]
if find(a) == find(b) {
return edge
}
parent[find(a)] = find(b)
}
return []
}
private func find(_ x: Int) -> Int {
if parent[x] != x {
parent[x] = find(parent[x])
}
return parent[x]
}
}
|